oninit()
, onslide()
and onpanelclick()
event handlers
oninit()
, onslide()
and onpanelclick()
are
three event handlers
you can define as parameters within your configuration code to perform your own action
when the
following three events happen:
- A Carousel Viewer has finished initializing on the page and ready for
user interaction-
oninit()
thenonslide()
each fires once. - User moves to a certain panel-
onslide()
is fired each time. - User clicks on a panel-
onpanelclick(target)
is fired and passed the actual element (target
) the user clicked on within the panel.
oninit()
event
To start out with, lets see how the oninit()
event handler
works:
<script type="text/javascript">
stepcarousel.setup({
galleryid: 'galleryC', //id of carousel DIV
beltclass: 'belt', //class of inner "belt" DIV containing all the panel DIVs
panelclass: 'panel', //class of panel DIVs each holding content
panelbehavior: {speed:300, wraparound:false, persist:true},
defaultbuttons: {enable: true, moveby: 1, leftnav: ['arrowl.gif', -10, 100],
rightnav: ['arrowr.gif', -10, 100]},
statusvars: ['imageA', 'imageB', 'imageC'], //register 3 variables that contain
current panel (start), current panel (last), and total panels
contenttype: ['inline'], //content setting ['inline'] or ['external',
'path_to_external_file']
oninit:function(){
isloaded=true
alert("Carousel has initialized")
}
})
</script>
All the above does is populate a variable and alert a message when the
Carousel has finished loading, but it shows how the oninit()
event
works nicely. Use it to do one time tasks once the Carousel has loaded, such as
declare variables, reference any of the Status Variables and do something with
them etc.
onslide()
event
The onslide()
event is fired whenever the user moves to a panel
within the Carousel, by calling stepTo()
or
stepBy()
. It is also fired 1 time after the Carousel has loaded
(same time as oninit()
). Use this event to perform tasks that
should be updated each time the Carousel moves. Lets say you've set up your Carousel Viewer to show one panel at a time,
and as each panel is shown, you wish to display additional text somewhere else
on the page describing that panel. I'll use the oninit()
event
handler to reference the DIV that will be showing the description, and onslide()
to
populate the DIV on the page with the related text each time the Carousel
slides:
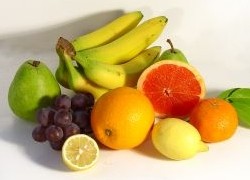
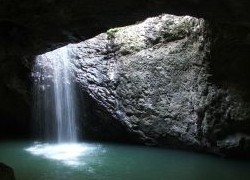
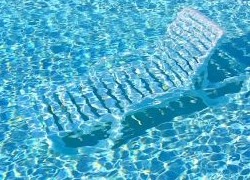
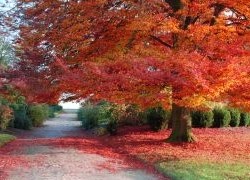
Here's how your configuration code would look like:
<script type="text/javascript">
var contentarray=new Array() //Array storing
the corresponding description for each Carousel panel
contentarray[0]="A fruit a day keeps the doctor away!"
contentarray[1]="Some day I will visit one of these lovely caves!"
contentarray[2]="Nothing beats floating around in the pool when it's hot"
contentarray[3]="Autumn, the season that just doesn't get no respect!"
stepcarousel.setup({
galleryid: 'galleryA', //id of carousel DIV
beltclass: 'belt', //class of inner "belt" DIV containing all the panel DIVs
panelclass: 'panel', //class of panel DIVs each holding content
panelbehavior: {speed:300, wraparound:false, persist:true},
defaultbuttons: {enable: true, moveby: 1, leftnav: ['arrowl.gif', -10, 100],
rightnav: ['arrowr.gif', -10, 100]},
statusvars: ['imageA', 'imageB', 'imageC'], //register 3 variables that contain
current panel (start), current panel (last), and total panels
contenttype: ['inline'], //content setting ['inline'] or ['external',
'path_to_external_file']
oninit:function(){
contentcontainer=document.getElementById('relatedcontent') //reference DIV
used to contain related content
},
onslide:function(){
//Update DIV with this panel's related content (notice imageA-1 as the
array index, as imageA starts at 1, while array at 0
contentcontainer.innerHTML=contentarray[imageA-1]
}
})
</script>
<div id="relatedcontent" style="margin-top: 5px; font: normal 13px Verdana">
</div>
"
"
While oninit()
and onslide()
both fire when the
Carousel Viewer first loads, I want to use oninit()
to perform one
time tasks like reference/ cache the DIV I'll be using later, instead of adding
it to
the onslide()
block, which is inefficient. Then, onslide()
is used to update the DIV with the corresponding description for each panel as
the viewer slides to it.
onpanelclick(target)
event
The onpanelclick(target)
is triggered whenever the user clicks
on a panel within a Carousel Viewer. The "target
" parameter of this
event contains a reference to the actual object clicked. In a panel where there
are images, text, and links, "target
" changes depending on
what was actually clicked on. In the below example, lets open any image links
clicked inside our panels in its own window:
<script type="text/javascript">
stepcarousel.setup({
galleryid: 'galleryB', //id of carousel DIV
beltclass: 'belt', //class of inner "belt" DIV containing all the panel DIVs
panelclass: 'panel', //class of panel DIVs each holding content
panelbehavior: {speed:300, wraparound:false, persist:true},
defaultbuttons: {enable: true, moveby: 1, leftnav: ['arrowl.gif', -10, 100],
rightnav: ['arrowr.gif', -10, 100]},
contenttype: ['inline'], //content setting ['inline'] or ['external',
'path_to_external_file']
onpanelclick:function(target){
if (target.tagName=="IMG" && target.parentNode.tagName=="A"){
window.open(target.parentNode.href, "", "width= 800px, height=700px,
scrollbars=1, left=100px, top=50px")
return false //cancel default action (load link in current window)
}
}
})
</script>
<div id="galleryB" class="stepcarousel">
<div class="belt">
<div class="panel">
<a href="http://en.wikipedia.org/wiki/Fruits"><img src="fruits.jpg" /></a>
</div>
<div class="panel">
<a href="http://en.wikipedia.org/wiki/Caves"><img src="cave.jpg" /></a>
</div>
<div class="panel">
<a href="http://en.wikipedia.org/wiki/Swimming_pool"><img src="pool.jpg" /></a>
</div>
<div class="panel">
<a href="http://en.wikipedia.org/wiki/Autumn"><img src="autumn.jpg" /></a>
</div>
The above illustrates a subtle but important point. Each of my panels above is a hyperlinked image, and in order to get each link to open in a new window using onpanelclick(target), the code looks like:
if (target.tagName=="IMG" &&
target.parentNode.tagName=="A"){
window.open(target.parentNode.href, "", "width= 800px, height=700px,
scrollbars=1, left=100px, top=50px")
return false //cancel default action (load link in current window)
}
Recall that "target
" always contain the target object the user
clicked on. In an image link, the target itself is the image (IMG
),
not the link, so our detection code has to be for an image (IMG
)
with its parent element being a link (A
), and if detected, load the
link in a new window.
Note: See
DOM
element properties for the properties that exist on the target
object.
Table Of Contents